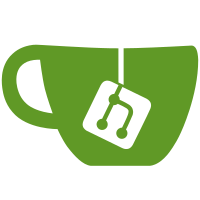
Both the robot and the base station had a lot of code that was identical, like data structs, constants, radio library etc. The chosen solution was to extract all shared code into a static library and link the station and robot against it.
27 lines
602 B
C
27 lines
602 B
C
#ifndef COMMON_H
|
|
#define COMMON_H
|
|
|
|
#include <stdint.h>
|
|
|
|
// Bit manioulation
|
|
#define BIT(mask) (1 << (mask))
|
|
#define SET(var, mask) ((var) |= (uint8_t)BIT(mask))
|
|
#define CLR(var, mask) ((var) &= (uint8_t)~(BIT(mask)))
|
|
#define CHK(var, mask) ((var) & (uint8_t)BIT(mask))
|
|
#define TOG(var, mask) ((var) ^= (uint8_t)BIT(mask))
|
|
|
|
// Maximum data size in bytes that can be sent/received with current nrf24L01+ lib
|
|
#define MAX_PAYLOAD_SIZE 32
|
|
|
|
enum return_codes {
|
|
R_OK,
|
|
R_PACKAGE_SIZE,
|
|
};
|
|
|
|
typedef struct {
|
|
double temp;
|
|
uint16_t distance;
|
|
} data_packet_t;
|
|
|
|
#endif /* COMMON_H */
|